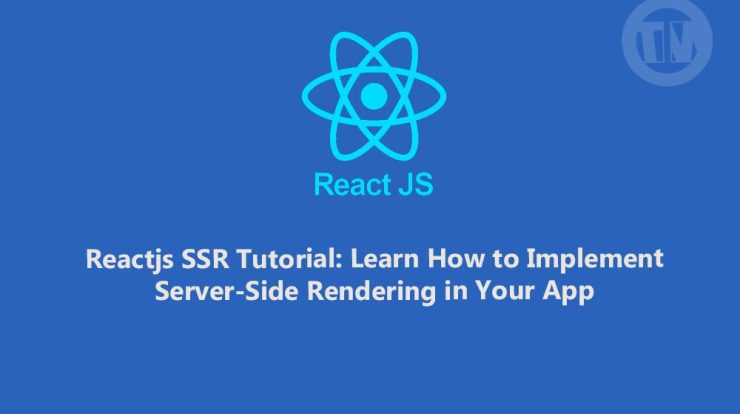
Are you looking for ways to improve the performance of your React application? Server-side rendering (SSR) can help you achieve faster page loads, better search engine optimization (SEO), and improved user experience. In this tutorial, we will walk you through the basics of React SSR and provide you with example code to get started.
What is Server-Side Rendering (SSR)?
Server-side rendering (SSR) is the process of rendering a web page on the server before sending it to the client’s browser. This is in contrast to client-side rendering (CSR), where the web page is generated on the client’s browser using JavaScript.
The main benefit of SSR is that it allows the client to receive a fully rendered page immediately, without waiting for the JavaScript to load and execute. This improves the user experience and can also help with SEO by providing search engines with a fully rendered page.
Why Use SSR with React?
React is a popular JavaScript library for building user interfaces. It uses a virtual DOM to efficiently update the UI when data changes. However, the initial render of a React app can be slow, especially on slow or unreliable networks. This is because the client has to download the JavaScript bundle, parse it, and then render the page.
SSR can help with this by rendering the initial HTML on the server and sending it to the client’s browser. This can reduce the time it takes for the page to load and make it more accessible to users on slower networks.
How to Implement SSR with React
Implementing SSR with React can be a complex process, but there are several tools and libraries available to help. Here are the basic steps involved:
- Create a server-side entry point
- Configure webpack for server-side rendering
- Use a server-side rendering framework (such as Next.js or Gatsby)
- Write server-side code to fetch data and render the page
- Update client-side code to handle hydration
Let’s take a closer look at each of these steps.
Step 1: Create a server-side entry point
To implement SSR with React, you need to create a server-side entry point that will render your React components. This file should import your React components and any required dependencies, such as Express.js or Koa.
// server.js import React from 'react'; import { renderToString } from 'react-dom/server'; import App from './App'; const express = require('express'); const app = express(); app.get('*', (req, res) => { const html = renderToString(<App />); res.send(html); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, we are using Express.js as our server-side framework and rendering our App component using renderToString from the react-dom/server package.
Step 2: Configure webpack for server-side rendering
Next, you need to configure webpack to bundle your server-side code. This can be done using the webpack-node-externals plugin, which tells webpack to exclude node_modules dependencies from the server-side bundle.
// webpack.config.js const nodeExternals = require('webpack-node-externals'); module.exports = { entry: './server.js', output: { filename: 'bundle.js', path: __dirname + '/dist', }, target: 'node', externals: [nodeExternals()], };
In this example, we are using the nodeExternals plugin to exclude node_modules dependencies from the server-side bundle.
Step 3: Use a server-side rendering framework
To simplify the process of implementing SSR with React, you can use a server-side rendering framework. Two popular options are Next.js and Gatsby.
Next.js is a framework that provides built-in support for SSR, automatic code splitting, and hot module replacement. It also includes features such as static site generation and API routes.
Gatsby is a static site generator that uses React and GraphQL. It includes features such as image optimization, code splitting, and pre-fetching data.
Both frameworks can be used to build fast and scalable React applications with SSR.
Step 4: Write server-side code to fetch data and render the page
To render a React component on the server, you need to provide it with the required data. This can be done using server-side code to fetch data from an API or database.
// server.js import React from 'react'; import { renderToString } from 'react-dom/server'; import App from './App'; import axios from 'axios'; const express = require('express'); const app = express(); app.get('*', async (req, res) => { const { data } = await axios.get('https://api.example.com/data'); const html = renderToString(<App data={data} />); res.send(html); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, we are using the axios library to fetch data from an API and passing it as a prop to our App component.
Step 5: Update client-side code to handle hydration
Once the server has rendered the initial HTML, the client-side code needs to take over and handle hydration. Hydration is the process of attaching event listeners and initializing the React app on the client’s browser.
// client.js import React from 'react'; import ReactDOM from 'react-dom'; import App from './App'; ReactDOM.hydrate(<App />, document.getElementById('root'));
In this example, we are using ReactDOM.hydrate to attach event listeners and initialize the React app on the client’s browser.
Example Code: React SSR with Next.js
Here is an example of implementing SSR with React using Next.js:
// pages/index.js import axios from 'axios'; function Home({ data }) { return ( <div> <h1>{data.title}</h1> <p>{data.body}</p> </div> ); } export async function getServerSideProps() { const { data } = await axios.get('https://jsonplaceholder.typicode.com/posts/1'); return { props: { data, }, }; } export default Home;
In this example, we are using Next.js to implement SSR and fetching data from an external API using axios. We are also using the getServerSideProps function to fetch data on the server and pass it as props to our Home component.
Conclusion
Server-side rendering is a powerful technique for improving the performance and SEO of your React application. By rendering the initial HTML on the server and sending it to the client’s browser, you can reduce the time it takes for your app to load and improve the user experience.
In this tutorial, we have walked you through the basics of React SSR and provided you with example code to get started. We hope that this will help you to implement SSR in your own React applications and improve their performance.
FAQs
- What is the difference between server-side rendering and client-side rendering? Server-side rendering (SSR) is the process of rendering a web page on the server before sending it to the client’s browser, while client-side rendering (CSR) is the process of rendering a web page on the client’s browser using JavaScript.
- Why should I use server-side rendering with React? Server-side rendering can improve the performance and SEO of your React application by reducing the time it takes for your app to load and providing search engines with easily crawlable HTML content.
- What server-side rendering frameworks are available for React? Two popular server-side rendering frameworks for React are Next.js and Gatsby.
- How can I fetch data for server-side rendering? You can fetch data using server-side code, such as Node.js, to make API requests or query a database.
- Can I use server-side rendering with other JavaScript frameworks besides React? Yes, server-side rendering is a technique that can be used with any JavaScript framework, including Angular and Vue.js.