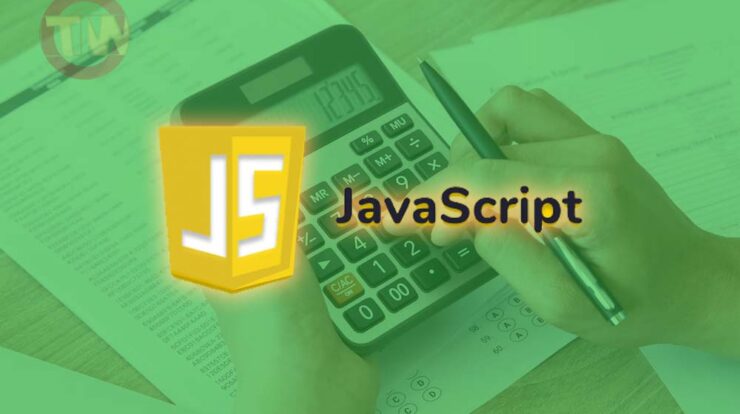
JavaScript is a popular programming language that is widely used in web development. One of the basic applications of JavaScript is the creation of a simple calculator. A simple calculator in JavaScript can be used to perform basic arithmetic operations such as addition, subtraction, multiplication, and division.
It can also be used to perform advanced operations such as square root, exponentiation, and logarithm. In this article, we will discuss how to download a simple calculator source code in JavaScript.
Why use a Simple Calculator in JavaScript?
There are several benefits of using a simple calculator in JavaScript. Firstly, it can help you to perform basic calculations quickly and easily. Secondly, it can be customized to meet your specific needs. For example, you can add or remove buttons depending on your requirements. Thirdly, it can be used as a learning tool to understand the basics of JavaScript programming. Finally, it can be integrated into your website or application to provide a user-friendly interface for performing calculations.
Simple Calculator in JavaScript Preview
How to Create a Simple Calculator in JavaScript
- Open Sublime text or your text editor
- Click file and select “save as” and named it “index.html“
- Then, click file and select “save as” and named it “app.js“ to make JavaScript file
- Last click “file” again and “save as” named it “style.css“ to make CSS file
- Download source code bellow and copy code to each of your files
Copy the code bellow for your html file
<html>
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
<link href="https://fonts.googleapis.com/css?family=Open+Sans:600,700" rel="stylesheet">
<title>A simple calculator</title>
</head>
<body>
<!-- div class -->
<div id="container">
<div id="calculator">
<div id="result">
<div id="history">
<p id="history-value"></p>
</div>
<div id="output">
<p id="output-values"></p>
</div>
</div>
<div id="keyboard">
<button class="operator" id="clear">C</button>
<button class="operator" id="backspace">CE</button>
<button class="operator" id="%">%</button>
<button class="operator" id="/">÷</button>
<button class="number" id="7">7</button>
<button class="number" id="8">8</button>
<button class="number" id="9">9</button>
<button class="operator" id="*">×</button>
<button class="number" id="4">4</button>
<button class="number" id="5">5</button>
<button class="number" id="6">6</button>
<button class="operator" id="-">-</button>
<button class="number" id="1">1</button>
<button class="number" id="2">2</button>
<button class="number" id="3">3</button>
<button class="operator" id="+">+</button>
<button class="empty" id="empty"></button>
<button class="number" id="0">0</button>
<button class="empty" id="empty"></button>
<button class="operator" id="=">=</button>
</div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Copy the code bellow for JavaScript module
function getHistory(){
return document.getElementById("history-value").innerText;
}
function printHistory(num){
document.getElementById("history-value").innerText=num;
}
function getOutput(){
return document.getElementById("output-values").innerText;
}
function printOutput(num){
if(num==""){
document.getElementById("output-values").innerText=num;
}
else{
document.getElementById("output-values").innerText=getFormattedNumber(num);
}
}
function getFormattedNumber(num){
if(num=="-"){
return "";
}
var n=Number(num);
var value= n.toLocaleString("en");
return value;
}
function reverseNumberFormat(num){
return Number(num.replace(/,/g, ''));
}
var operator = document.getElementsByClassName("operator");
for( var i=0;i<operator.length;i++){
operator[i].addEventListener('click',function(){
if(this.id=="clear"){
printHistory("");
printOutput("");
}
else if(this.id=="backspace"){
var
output=reverseNumberFormat(getOutput()).toString();
if(output){//if output has a value
output= output.substr(0,output.length-1);
printOutput(output);
}
}
else{
var output = getOutput();
var history = getHistory();
if(output==""&&history!=""){
if(isNaN(history[history.length-1])){
history=history.substr(0,history.length-1);
}
}
if(output!="" || history!=""){
output= output==""?
output:reverseNumberFormat(output);
history=history+output;
if(this.id=="="){
var result= eval(history);
printOutput(result);
printHistory("");
}
else{
history=history+this.id;
printHistory(history);
printOutput("");
}
}
}
});
}
var number = document.getElementsByClassName("number");
for(var i=0;i<number.length;i++){
number[i].addEventListener('click',function(){
var output=reverseNumberFormat(getOutput());
if(output!=NaN){
output=output+this.id;
printOutput(output);
}
});
}
Copy the code bellow for CSS style
body{
font-family: 'Open Sans',sans-serif;
background-color:black;
}
#container{
width: 1000px;
height: 550px;
background-image:linear-gradient(rgba(0,0,0,0.6),rgba(0,0,0,0.6)), url(Sugar-Excipients.jpg);
margin: 20px auto;
}
#calculator{
width: 320px;
height: 520px;
background-color: #eaedef;
margin: 0 auto;
top: 20px;
position: relative;
border-radius: 5px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0.2), 0 6px 20px 0 rgba(0,0,0,0.19);
}
#result{
height: 120px;
}
#history{
text-align: right;
height: 20px;
margin: 0 20px;
padding-top: 20px;
font-size: 15px;
color: #919191;
}
#output{
text-align: right;
height: 60px;
margin: 10px 20px;
font-size: 30px;
}
#keyboard{
height: 400px;
}
.operator, .number, .empty{
width: 50px;
height: 50px;
margin: 15px;
float: left;
border-radius: 50%;
border-width: 0;
font-size: 15px;
font-weight: bold;
}
.number, .empty{
background-color: #eaedef;
}
.operator{
background-color:lightgrey;
}
.number, .operator{
cursor: pointer;
}
.number:active, .operator:active{
font-size: 13px;
}
.number:focus, .operator:focus, .empty:focus{
outline: 0;
}
button:nth-child(4){
font-size: 20px;
background-color: #20b2aa;
}
button:nth-child(8){
font-size: 20px;
background-color: #ffa500;
}
button:nth-child(12){
font-size: 20px;
background-color: #f08080;
}
button:nth-child(16){
font-size: 20px;
background-color: #7d93e0;
}
button:nth-child(20){
font-size: 20px;
background-color: #9477af;
}
Download Simple Calculator Source Code in JavaScript
ABOUT PROJECT | PROJECT DETAILS |
---|---|
Project Name : | Calculator In JavaScript |
Project Platform : | JavaScript |
Programming Language Used: | JavaScript, HTML, and CSS |
IDE Tool (Recommended): | Sublime |
Project Type : | Web Application |
Database: | None |
To download the source code for Simple Calculator project in JavaScript, simply click on this link download source code of Simple Calculator in JavaScript.
Conclusion
Simple calculator in JavaScript can be a useful tool for performing basic arithmetic operations quickly and easily. By downloading a simple calculator source code in JavaScript, you can customize the calculator to meet your specific needs. Whether you want to add or remove buttons, change the color scheme, or modify the functionality, JavaScript provides the flexibility and versatility to do so.
With the popularity of JavaScript in web development, a simple calculator in JavaScript can also be integrated into your website or application to provide a user-friendly interface for performing calculations. So, go ahead and download a simple calculator source code in JavaScript today to start exploring the possibilities!