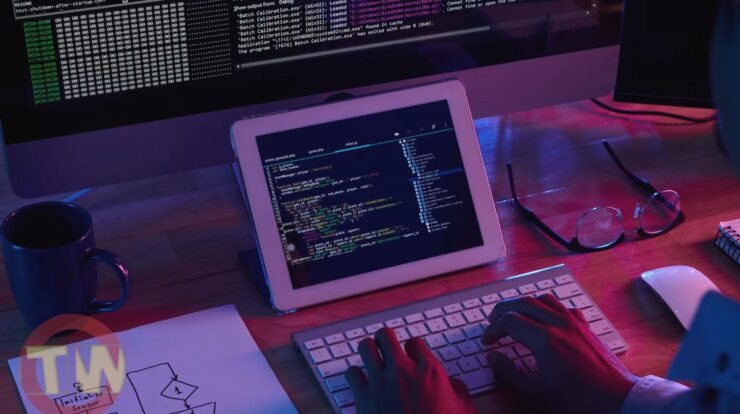
JavaScript is a widely used programming language that is essential for creating dynamic and interactive web pages. It is used in web development, mobile app development, game development, and much more. In this article, we will discuss JavaScript and its importance, as well as provide a step-by-step guide on how to download a file using JavaScript.
Understanding JavaScript
JavaScript is a high-level, object-oriented programming language that is commonly used to create dynamic web pages. It is a scripting language that runs on the client-side, which means that it is executed by the user’s web browser, rather than on the web server. JavaScript is a versatile language that can be used for a wide variety of applications, including creating interactive user interfaces, validating user input, and manipulating data.
Advantages of JavaScript
One of the key advantages of JavaScript is its versatility. It can be used for a wide variety of applications, from simple web forms to complex web applications. It is also relatively easy to learn, especially for those who are already familiar with other programming languages. Other advantages of JavaScript include its speed and the fact that it is supported by all major web browsers.
Disadvantages of JavaScript
One of the main disadvantages of JavaScript is that it can be easily disabled or blocked by the user. This can lead to issues with the functionality of web pages that rely heavily on JavaScript. Additionally, JavaScript can be prone to errors and bugs, especially when it is used to create complex applications.
How to Download a File Using JavaScript
JavaScript can be used to download files from a web page. This is a useful feature for web developers who want to provide their users with the ability to download files from their web pages. Here is a step-by-step guide on how to download a file using JavaScript:
- First, create an HTML button or link that will trigger the download process when clicked.
- Next, add an event listener to the button or link that will execute the download process when clicked.
- In the event listener, create a new XMLHttpRequest object, which is used to communicate with the server and retrieve the file.
- Use the XMLHttpRequest object to send a request to the server to retrieve the file.
- When the file is retrieved, use the JavaScript Blob object to create a new file object that contains the downloaded file.
- Finally, use the JavaScript URL object to create a URL that can be used to download the file.
Here is an example of the code that can be used to download a file using JavaScript:
function downloadFile() { var xhr = new XMLHttpRequest(); xhr.open('GET', 'https://example.com/file.pdf', true); xhr.responseType = 'blob'; xhr.onload = function(e) { if (this.status == 200) { var blob = new Blob([this.response], {type: 'application/pdf'}); var url = URL.createObjectURL(blob); var a = document.createElement('a'); a.href = url; a.download = 'file.pdf'; document.body.appendChild(a); a.click(); document.body.removeChild(a); } }; xhr.send(); }
Conclusion
JavaScript is an essential programming language for web development, mobile app development, and much more. Its versatility and ease of use make it a popular choice for developers who want to create dynamic and interactive web pages.
In this article, we have discussed the importance of JavaScript and provided a step-by-step guide on how to download a file using JavaScript. We hope that this guide has been useful to you and that you have gained a better understanding of the power of JavaScript.