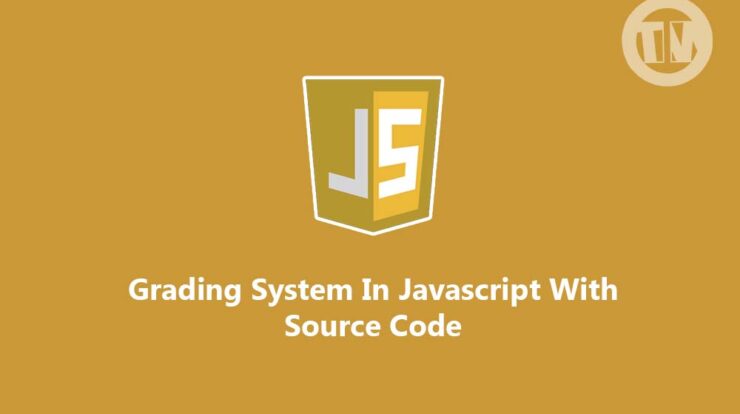
Are you looking for a grading system source code written in Javascript? Look no further! In this article, we will discuss what a grading system is, why it is important, and how to download a grading system source code in Javascript.
What is Grading System?
A grading system is a method of evaluating a student’s performance in a course or subject. It is used to assess the quality of work submitted by a student and to assign a corresponding letter grade. Grading systems are used in educational institutions all over the world, from elementary schools to universities.
Grading systems are important because they provide a way to measure a student’s progress and achievement in a course or subject. They help educators to identify areas where a student may need additional support or instruction. Grading systems also serve as a means of communication between educators and students, providing feedback on performance and areas for improvement.
Why build Grading System using JavaScript?
Javascript is a popular programming language used in web development. It is a versatile language that can be used to create dynamic and interactive web pages. Grading systems built using Javascript offer several advantages over traditional grading systems.
Firstly, Javascript grading systems are more flexible than traditional grading systems. They can be customized to fit the specific needs of an institution or teacher. Javascript grading systems can be modified to accommodate different grading scales, weighting schemes, and reporting formats.
Secondly, Javascript grading systems are more interactive than traditional grading systems. They can provide students with real-time feedback on their performance and progress. Javascript grading systems can also incorporate multimedia elements such as videos and animations to enhance the learning experience.
Lastly, Javascript grading systems are more accessible than traditional grading systems. They can be accessed from any device with an internet connection, including smartphones and tablets. This makes it easier for students to stay up-to-date with their grades and progress.
Grading System Project Preview
How to Create a Grading System project in JavaScript
- Download project file, scroll down to get the download link
- After the download source code is complete, extract the file
- Open Sublime Text or your text editor
- Then, create a html file and save as index.html
- Copy the code we given bellow
Code for the html module
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Grading System in Javascript</title> </head> <body style="background-color: skyblue"> <center> <h1 style="margin-top: 10px">Grading System in Javascript (IT SOURCECODE)</h1> </center> <div id="main"> <div id="head"><h1>Grading System In JS</h1></div> <label>English</label><input type="text" id="eng"><br> <label>Maths</label><input type="text" id="mat"><br> <label>Physics</label><input type="text" id="phy"><br> <label>Chemistry</label><input type="text" id="chm"><br> <label>Computer</label><input type="text" id="cmp"><br> <br> <button class="btn1" onclick="Total()">Total</button> <button class="btn2" onclick="Average()">Average</button> <button class="btn3" onclick="Grade()">Grade</button> </div> <div id="result"> <div id="head1"><h1>Total</h1></div> <div id="total"> </div> <div id="head2"><h1>Average</h1></div> <div id="avg"> </div> <div id="head3"><h1>Grade</h1></div> <div id="grade"> </div> </div> </body> </html>
Code for CSS module
<style> *{ padding: 0; margin: 0; box-sizing: border-box; } body{ background-size: cover; } #main { width: 40%; height: 500px; background-color: white; text-align: center; float: left; margin: 40px 10px 0 150px; } #head{ background-color: black; width: 100%; height: 55px; color: white; padding: 10px; margin-bottom: 50px; } #main input{ height: 30px; width: 45%; margin-bottom: 10px; font-size: 20px; } #main label{ font-size: 23px; padding-right: 50px; margin-bottom: 10px; } .btn1{ outline: none; border: none; width: 14%; height: 40px; margin-right: 30px; margin-top: 50px; font-size: 22px; background-color: teal; } .btn2{ outline: none; border: none; width: 20%; height: 40px; font-size: 22px; background-color: teal; margin-right: 30px; margin-top: 50px; } .btn3 { outline: none; border: none; width: 14%; height: 40px; background-color: teal; margin-top: 50px; font-size: 22px; } #result { height: 500px; width: 30%; float: left; margin-top: 40px; background-color: white; } #head1, #head2, #head3 { background-color:black; width: 100%; height: 45px; color: white; text-align: center; } #total { height: 200px; width: 100%; padding: 20px; background-color: white; font-size: 19px; font-weight: 600; } #grade { height: 60px; width: 100%; padding: 20px; font-size: 19px; font-weight: 600; background-color: white; } #avg{ height: 80px; width: 100%; padding: 20px; font-size: 19px; font-weight: 600; background-color: white; } </style>
Code for the JavaScript module
<script> function Total(){ var sub1 = parseInt(document.getElementById("eng").value); var sub2 = parseInt(document.getElementById("mat").value); var sub3 = parseInt(document.getElementById("phy").value); var sub4 = parseInt(document.getElementById("chm").value); var sub5 = parseInt(document.getElementById("cmp").value); if(sub1>100 || sub2>100 || sub3>100 || sub4>100 || sub5>100 ) { alert("Please Enter Marks in range of 100"); } else { var total= sub1 + sub2 + sub3 + sub4 + sub5; document.getElementById("total").innerHTML = "English Marks :"+sub1+"<br> Maths Marks: "+sub2+"<br> Physics Marks: "+sub3+"<br> Chemistry Marks: "+sub4+"<br> Computer Marks: "+sub5+"<br> Total Marks: "+total; } } function Average(){ var sub1 = parseInt(document.getElementById("eng").value); var sub2 = parseInt(document.getElementById("mat").value); var sub3 = parseInt(document.getElementById("phy").value); var sub4 = parseInt(document.getElementById("chm").value); var sub5 = parseInt(document.getElementById("cmp").value); if(sub1>100 || sub2>100 || sub3>100 || sub4>100 || sub5>100 ) { alert("Please Enter Marks in range of 100"); } else { var total= sub1 + sub2 + sub3 + sub4 + sub5; var avg=total/5; document.getElementById("avg").innerHTML="Your Average marks are: "+avg; } } function Grade(){ var sub1 = parseInt(document.getElementById("eng").value); var sub2 = parseInt(document.getElementById("mat").value); var sub3 = parseInt(document.getElementById("phy").value); var sub4 = parseInt(document.getElementById("chm").value); var sub5 = parseInt(document.getElementById("cmp").value); if(sub1>100 || sub2>100 || sub3>100 || sub4>100 || sub5>100 ) { alert("Please Enter Marks in range of 100"); }else { var total= sub1 + sub2 + sub3 + sub4 + sub5; var avg=total/5; if(avg>=80 && avg<=100) { document.getElementById("grade").innerHTML="You Got A+ Grade"; } else if(avg>=75 && avg<=80) { document.getElementById("grade").innerHTML="You Got A+ Grade"; } else if(avg>=70 && avg<=75) { document.getElementById("grade").innerHTML="You Got A Grade"; } else if(avg>=65 && avg<=70) { document.getElementById("grade").innerHTML="You Got B Grade"; } else if(avg>=50 && avg<=60) { document.getElementById("grade").innerHTML="You Got C Grade"; } else if(avg>=40 && avg<=50) { document.getElementById("grade").innerHTML="You Got C Grade"; } else { document.getElementById("grade").innerHTML="You Got F Grade"; } } } </script>
Download Grading System Source Code In Javascript
ABOUT PROJECT | PROJECT DETAILS |
---|---|
Project Name : | Grading System In Javascript |
Project Platform : | JavaScript |
Programming Language Used: | php,javascript,html,css |
IDE Tool (Recommended): | Sublime |
Project Type : | Web Application |
Database: | None |
To download the source code for Grading System project in JavaScript, simply click on this link download Grading System in JavaScript.
Conclusion
a grading system is an essential tool for assessing student performance and progress. Building grading systems using Javascript offers several advantages over traditional grading systems, including flexibility, interactivity, and accessibility. Downloading grading system source code in Javascript can save time and effort in building a grading system from scratch.