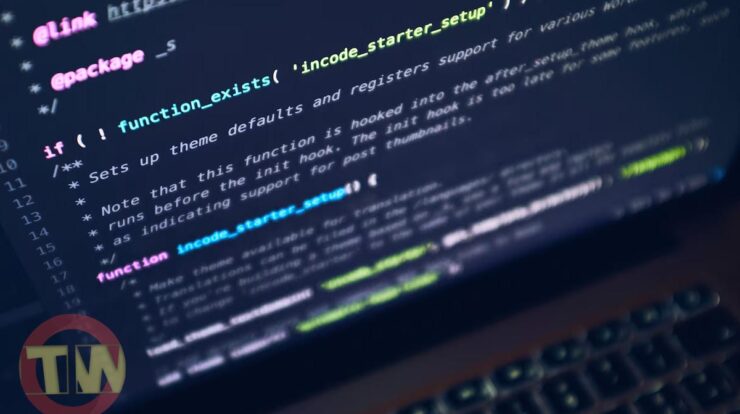
Are you looking for a way to enhance your website’s user experience with voice interaction? Or perhaps you want to create a more accessible website for people with visual impairments? Whatever your reason, incorporating a text-to-speech feature using JavaScript is a great way to achieve these goals. In this article, we will walk you through the steps to create a text-to-speech feature using JavaScript.
What is Text-to-Speech?
Text-to-speech, or TTS, is a technology that allows computers to read text aloud. TTS can be used to enhance the user experience of your website or application, especially for those who have difficulty reading or those who prefer listening to content. TTS is also helpful for people with visual impairments or reading difficulties.
Speech to Text in JavaScript with Source Code Steps on How to Create a Project
Here’s the Steps on how to create a JavaScript speech to text with Source Code
- Open Sublime Text
- Click “file” and select “save as” and named it “index.html“ to create a HTML file.
- Create a JavaScript file, click “file” and select “save as” and named it “script.js“.
- Click “file” and select “save as” and named it “style.css“ to create a CSS file.
- Last, copy all the code given below and paste to your different file created (HTML, Javascript and CSS).
Code for the index.html in Speech Text Reader in JavaScript
Copy the code below into the HTML file you just created, which is the code contains the interface of the application. This code will show the form that will be use in html.
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <title>Speech Text Reader</title> <meta name="description" content="" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <link rel="stylesheet" href="style.css" /> </head> <body> <div class="container"> <h1>Speech Text Reader</h1> <button id="toggle" class="btn btn-toggle">Toggle text box</button> <div id="text-box" class="text-box"> <div id="close" class="close">X</div> <h3>Choose Choice</h3> <select name="" id="voice"></select> <textarea id="text" placeholder="Enter text to read..."></textarea> <button id="read" class="btn">Read text</button> </div> <main></main> </div> <script src="script.js" async defer></script> </body> </html>
Code for the style.css in Speech Text Reader in JavaScript
Copy the code below into the CSS file you just created, which is the code contains the interface design of the application. This code will show the form that will be use for designing.
@import url("https://fonts.googleapis.com/css2?family=Righteous&display=swap"); * { box-sizing: border-box; } body { font-family: "Righteous", cursive; background-color: #e8e8e8; color: #222831; min-height: 100vh; margin: 0; } h1 { text-align: center; color: white; } .container { margin: auto; padding: 20px; background-color: black; } .btn { cursor: pointer; background-color: blue; color: white; border: 0; border-radius: 5px; padding: 10px 15px; } .btn:active { transform: scale(0.9); } .btn:focus, select:focus { outline: 0; } .btn-toggle { display: block; margin: auto; margin-bottom: 20px; } .text-box { width: 70%; position: absolute; top: 30%; left: 50%; transform: translate(-50%, -800px); background-color: #00cc00; color: #e8e8e8; padding: 20px; border-radius: 5px; transition: all 1s ease-in-out; } .text-box.show { transform: translate(-50%, 0); } .text-box select { background-color: #0033cc; border: 0; color: #e8e8e8; font-size: 12px; height: 30px; width: 100%; } .text-box textarea { border: 1px solid #30475e; border-radius: 5px; font-size: 16px; padding: 8px; margin: 15px 0; width: 100%; height: 150px; } .text-box .btn { position: absolute; right: 30px; bottom: 50px; } .text-box .close { float: right; text-align: right; cursor: pointer; } main { display: grid; grid-template-columns: repeat(4, 1fr); gap: 10px; } .box { box-shadow: 0 2px 10px rgba(0, 0, 0, 0.2); border-radius: 5px; cursor: pointer; display: flex; flex-direction: column; overflow: hidden; transition: box-shadow 0.2s ease-out; } .box.active { box-shadow: 0 0 10px 5px #f05454; } .box img { width: 100%; object-fit: cover; height: 200px; } .box .info { background-color: blue; color: white; font-size: 18px; letter-spacing: 1px; text-transform: uppercase; margin: 0; padding: 10px; text-align: center; height: 100%; } @media (max-width: 1100px) { main { grid-template-columns: repeat(3, 1fr); } } @media (max-width: 760px) { main { grid-template-columns: repeat(2, 1fr); } } @media (max-width: 500px) { main { grid-template-columns: 1fr; } }
Code for the script.js in Speech Text Reader in JavaScript
Copy the code below into the JavaScript file you just created, which is the code contains the interface function of the application. This code will show the function of the form that will be use in html.
const main = document.querySelector("main"); const voiceSelect = document.getElementById("voice"); const textarea = document.getElementById("text"); const readBtn = document.getElementById("read"); const toggleBtn = document.getElementById("toggle"); const closeBtn = document.getElementById("close"); const data = [ { image: "./images/australia.png", text: "Australia", }, { image: "./images/china.jpg", text: "China", }, { image: "./images/india.jpg", text: "india", }, { image: "./images/japan.png", text: "Japan", }, { image: "./images/philippines.png", text: "philippines", }, { image: "./images/russia.jpg", text: "Russia", }, { image: "./images/southkorea.png", text: "South Korea", }, { image: "./images/usa.png", text: "United States of America", }, ]; data.forEach(createBox); //create box function createBox(item) { const box = document.createElement("div"); const { image, text } = item; box.classList.add("box"); box.innerHTML = ` <img src="${image}" alt="${text}" /> <p class="info">${text}</p> `; box.addEventListener("click", () => { setTextMessage(text); speakText(); box.classList.add("active"); setTimeout(() => box.classList.remove("active"), 800); }); main.appendChild(box); } const message = new SpeechSynthesisUtterance(); function setTextMessage(text) { message.text = text; } function speakText() { speechSynthesis.speak(message); } let voices = []; function getVoice() { voices = speechSynthesis.getVoices(); voices.forEach((voice) => { const option = document.createElement("option"); option.value = voice.name; option.innerText = `${voice.name} ${voice.lang}`; voiceSelect.appendChild(option); }); } speechSynthesis.addEventListener("voiceschanged", getVoice); getVoice(); toggleBtn.addEventListener("click", () => document.getElementById("text-box").classList.toggle("show") ); closeBtn.addEventListener("click", () => document.getElementById("text-box").classList.remove("show") ); function setVoice(e) { message.voice = voices.find((voice) => voice.name === e.target.value); } voiceSelect.addEventListener("change", setVoice); readBtn.addEventListener("click", () => { setTextMessage(textarea.value); speakText(); });