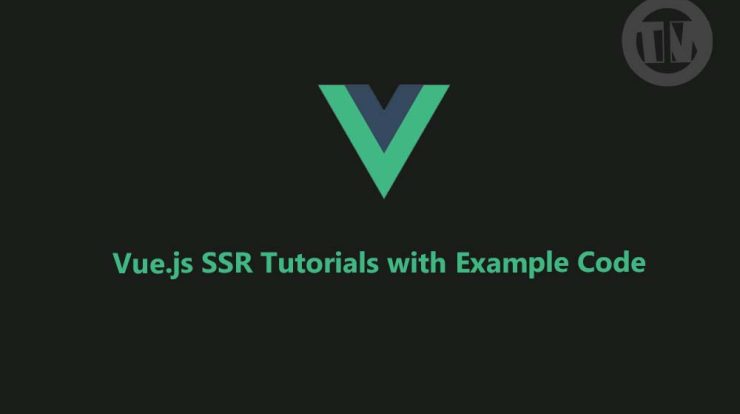
Vue.js is a popular JavaScript framework that enables developers to build interactive user interfaces and single-page applications (SPAs). Server-side rendering (SSR) is a technique that can be used to improve the performance of Vue.js applications. In this tutorial, we will explore Vue.js SSR and provide example code to help you implement it in your own projects.
What is Vue.js SSR?
Server-side rendering (SSR) is a technique that involves rendering web pages on the server before they are sent to the client. Vue.js SSR is the implementation of this technique in Vue.js applications. With SSR, the initial page load is faster because the server renders the HTML, CSS, and JavaScript for the page before it is sent to the client. This can improve the user experience by reducing the time it takes for the page to load.
Benefits of Vue.js SSR
There are several benefits of using Vue.js SSR, including:
- Improved performance: SSR can improve the performance of Vue.js applications by reducing the time it takes for the initial page load.
- Better SEO: SSR can improve the search engine optimization (SEO) of Vue.js applications by making them more easily indexable by search engines.
- Improved user experience: SSR can improve the user experience of Vue.js applications by reducing the time it takes for the page to load.
Getting Started with Vue.js SSR
To get started with Vue.js SSR, you will need to install a few dependencies. You will need Node.js and npm installed on your machine. You will also need to install the following packages:
- Vue.js: This is the main Vue.js package.
- Vue-server-renderer: This is the package that enables SSR in Vue.js applications.
Once you have installed these dependencies, you can start implementing SSR in your Vue.js application.
Implementing Vue.js SSR
To implement Vue.js SSR, you will need to create a server-side entry point for your application. This entry point should import your Vue.js application and render it using the Vue-server-renderer package.
Here is an example server-side entry point for a Vue.js application:
const express = require('express') const app = express() const Vue = require('vue') const serverRenderer = require('vue-server-renderer').createRenderer() app.get('/', (req, res) => { const vm = new Vue({ template: '<div>Hello, {{ name }}!</div>', data: { name: 'World' } }) serverRenderer.renderToString(vm, (err, html) => { if (err) { console.error(err) res.status(500).end('Internal Server Error') return } res.end(html) }) }) app.listen(3000, () => { console.log('Server started on port 3000') })
This entry point creates a new Vue instance with a simple template and data object. It then uses the Vue-server-renderer package to render the Vue instance to HTML. Finally, it sends the rendered HTML to the client.
Example Code for Vue.js SSR
Here is an example of a Vue.js component that can be used with SSR:
<template> <div> <h1>{{ title }}</h1> <p>{{ body }}</p> </div> </template> <script> export default { name: 'ExampleComponent', props: { title: String, body: String } } </script>
To render this component on the server using SSR, you would need to create a new Vue instance with this component as the root component. Here is an example of how to do that:
const vm = new
Example Code for Vue.js SSR (Continued)
const Vue = require('vue') const ExampleComponent = require('./ExampleComponent.vue') const serverRenderer = require('vue-server-renderer').createRenderer() const app = new Vue({ render: h => h(ExampleComponent, { props: { title: 'Example Title', body: 'Example Body' } }) }) serverRenderer.renderToString(app, (err, html) => { if (err) { console.error(err) return } console.log(html) })
This example creates a new Vue instance with the ExampleComponent
as the root component. It uses the renderToString
method of the vue-server-renderer
package to render the Vue instance to HTML.
Conclusion
Vue.js SSR is a powerful technique that can improve the performance and user experience of Vue.js applications. By rendering web pages on the server before they are sent to the client, SSR can reduce the time it takes for the initial page load and improve the search engine optimization of Vue.js applications. With the help of the example code provided in this tutorial, you can start implementing Vue.js SSR in your own projects.
FAQs
What is the difference between client-side rendering and server-side rendering?
Client-side rendering involves rendering web pages on the client-side (i.e., in the user’s web browser) using JavaScript. Server-side rendering involves rendering web pages on the server before they are sent to the client.
How does Vue.js SSR improve the performance of web applications?
Vue.js SSR can improve the performance of web applications by reducing the time it takes for the initial page load. By rendering web pages on the server before they are sent to the client, SSR can reduce the amount of work that the client’s web browser has to do.
Can Vue.js SSR be used with other JavaScript frameworks?
Yes, Vue.js SSR can be used with other JavaScript frameworks. However, the implementation may differ depending on the framework.
Does Vue.js SSR require a server-side runtime environment?
Yes, Vue.js SSR requires a server-side runtime environment such as Node.js.
Is Vue.js SSR suitable for all types of web applications?
Vue.js SSR is suitable for most types of web applications. However, it may not be suitable for applications that require a high level of interactivity or real-time updates.